Python Master Mind Game
Self-Paced Projects
Fee: ₹2,500
Mastermind is a classic code-breaking game for two players. One player, the code-maker, creates a secret code, and the other player, the code-breaker, tries to guess the code. The code is represented by a sequence of colored pegs, and the code-breaker must deduce both the correct colors and their positions based on feedback provided after each guess.
The code-maker creates a secret code consisting of a sequence of colored pegs. The number of pegs in the code and the range of possible colors are predetermined. The code-breaker attempts to guess the secret code.
After each guess, the code-maker provides feedback in the form of black and white pegs:
A black peg indicates a correct color in the correct position. A white peg indicates a correct color in the wrong position. The code-breaker continues guessing until they either correctly guess the code or reach a maximum number of guesses.
Objectives
Secret Code Generation: The codemaker generates a secret code consisting of a sequence of colored pegs or digits. The length of the code and the range of possible colors or digits are predetermined. Codebreaker's Guesses: The codebreaker makes a series of guesses to determine the secret code. After each guess, the codemaker provides feedback to indicate how close the guess is to the actual code. Feedback Mechanism: The feedback typically consists of clues such as the number of correct colors in the correct positions (black pegs) and the number of correct colors in the wrong positions (white pegs). Limited Attempts: The codebreaker has a limited number of attempts to guess the secret code correctly. The game ends when the codebreaker either guesses the correct code or exhausts all attempts. User Interface: Provide a user-friendly interface for inputting guesses and displaying feedback. This could be a text-based interface in the console or a graphical user interface (GUI) using libraries like Tkinter or Pygame. Scoring and Winning: You can implement scoring mechanisms to keep track of the number of attempts made by the codebreaker. The codebreaker wins if they guess the code within the specified number of attempts. By achieving these objectives, you'll create an engaging and interactive Mastermind game that challenges players' logic and deduction skills.
Your Python program should include the following functionalities:
Randomly generate the secret code.
Allow the player to input their guesses.
Provide feedback to the player after each guess.
Keep track of the number of attempts remaining.
End the game when the player correctly guesses the code or runs out of attempts.
Optionally, allow the player to choose the number of pegs in the code, the number of colors available, and the number of attempts allowed.
Your program should provide a clear and intuitive interface for the player to interact with. Additionally, it should handle inputs gracefully, providing helpful prompts and error messages where necessary.
What Will You Learn
Creating a Mastermind game using Python can be a fun and rewarding project! Here's what you can expect from this project:Game Logic Implementation: You'll need to implement the game's logic, including generating a random secret code for the player to guess and handling the player's guesses.
Skills you will gain
Curriculum
-
Project's Prelude
-
Project's Problem Description
-
Project's Problem Statement
-
Project's Objective
-
Project's Expected Features
-
High level Architecture and workflow and Technology
Other Details
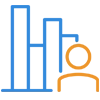

Associated Courses



